객체포인터와 객체배열, 객체의 동적생성
1. 객체포인터
(1) 객체 포인터 변수선언방법
Circle *p; //Circle 타입에 대한 포인터변수p
(2) 포인터변수에 주소지정방법
p = &donut; //도넛객체의 주소저장
Circle* p = &donut; //포인터 변수 선언시 주소로 초기화 가능
(3) 포인터를 이용한 객체 멤버접근
d = donut.getArea(); // 객체 이름으로 멤버를 접근함
d = p->getArea(); // 객체 포인터로 멤버를 접근할때는 ->를 쓴다
또는
d = (*p).getArea(); // 괄호를 넣어서 .을 쓸수도 있다.
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12
2, 객체배열
(1) 객체 배열 선언방법
Circle circleArray[3]; // Circle 객체의 배열 circleArray 를 선언
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12ㅇ
(2) 객체 배열을 이용한 멤버접근
circleArray[0].setRadius(10);
circleArray[1].setRadius(20);
circleArray[2].setRadius(30);
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12
(3) 객체배열 소멸순서
circleArrat[2] 소멸자 실행 -> circleArrat[1] 소멸자 실행 -> circleArrat[0] 소멸자 실행 (생성자 역순으로 소멸)
객체배열도 생성자 역순으로 소멸됨
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12
3. 정적할당 개념
프로그램 실행 전에 미리 할당 받는것으로 Java의 경우 static이 정적에 해당됨
stack과 data 영역에 컴파일시 할당되어야 할 메모리들로 , 전역변수 지역변수가 정적부분에 해당됨
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12
4. 동적할당개념
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12
5. 변수, 배열, 객체, 객체배열 동적할당과 소멸 코드설명 (수업시간 칠판내용)
[1]변수
할당
int *p = new int(5);
반환
delete p;
[2]배열
할당
int *p = new int[5];
int *p = new int[5](30); -> 오류, 크기값 지정 못함.
[] - 크기
() - 크기값
반환
delete [] p;
[3]객체
할당
Circle *p = new Circle(30);
반환
delete p;
-생성 순서와 상관없이 삭제할 순서 지정이 가능
[4]객체배열
할당
Circle *p = new Circle[3]
반환
delete [] p;
-삭제 순서는 생성된 순서 역순
크기값을 지정할때는 setRadius() 함수를 사용
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12
6. 메모리 누수현상이 발생하는 이유
동적으로 할당받은 메모리의 주소를 잃어버려 힙에 반환할 수 없게 되면 메모리 누수가 발생함
메모리 누수가 계속 발생하여 힙의 크기가 줄어들게 되면, 실행중에 메모리를 할당받을 수 없는 상황이 초래됨
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12
7. this가 필요한 경우
this는 객체 자신에 대한 포인터으로 각 객체 속의 this는 다른 객체 속의 this와 서로 다른 포인터임을 알리기 위해서 사용된다.
멤버 변수의 이름과 동일한 이름으로 매개 변수 이름을 짓고자 하는 경우와
객체의 멤버 함수에서 객체 자신의 주소를 리턴 할 때 this는 반드시 필요하다.
[출처] 4. 객체포인터와 객체배열, 객체의 동적생성 |작성자 jklj12
8. string 클래스를 이용한 문자열 예제(11가지, 수업시간 실습예제)
[1]문자열 치환
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
string a="Java", b="C++";
cout << "a = " << a << endl;
a=b;
cout << "a = " << a << endl;
}
[2]문자열 비교( compare() 함수)
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
string name="Kitae";
string alias="Kito";
int res=name.compare(alias); // name과 alias를 비교
if(res==0)
cout << "두 문자열이 같다";
else if(res<0)
cout << name << " < " << alias << endl; // name이 앞
else
cout << alias << " < " << name << endl; // name이 뒤
}
[3]문자열 연결(append() 함수)
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
string a("I");
a.append(" love"); //문자열연결
cout << a << endl;
}
[4]문자열 삽입(insert() 함수)
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
cout << "012345678901234567890" << endl;
string a("I love C++");
a.insert(2, "really ");
cout << a << endl;
}
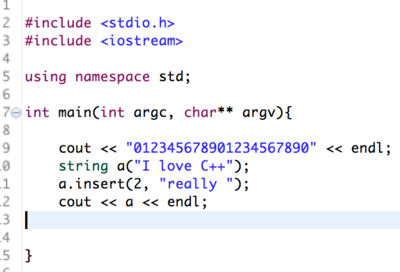
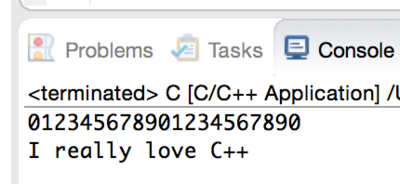
[5]문자열 길이(Length() 함수)
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
string a("I love C++");
int length=a.length();
int size=a.size();
int capacity=a.capacity();
cout << "length = " << length << ", size = "
<< size << ", capacity = " << capacity << endl;
}
[6]문자열 삭제(clear() 함수)
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
cout << "012345678901234567890" << endl;
string a("I love C++");
cout << a << endl;
a.erase(0,7); // erase(시작위치, 삭제할 갯수)
cout << " a = " << a << endl;
a.clear();
cout << " a = " << a << endl;
}
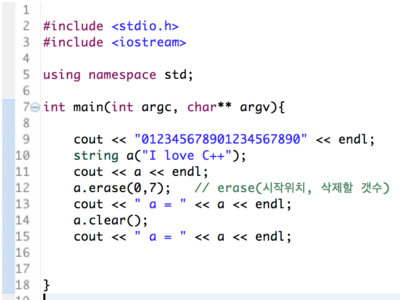
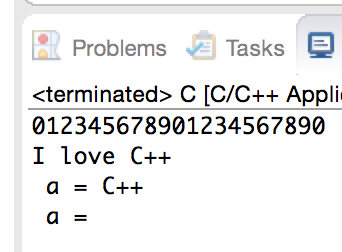
[7]문자열 일부분 발췌(잘라내는 것)(substr() 함수)
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
cout << "012345678901234567890" << endl;
string b="I love C++";
cout << b << endl;
string c=b.substr(2,4); // substr(시작위치, 갯수)
string d=b.substr(2); // substr(시작위치) -> 시작위치 ~ 끝까지 추출함
cout << "c = " << c << endl << "d = " << d << endl;
}
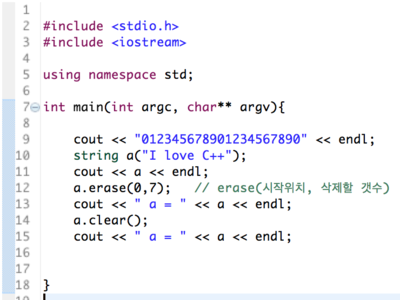
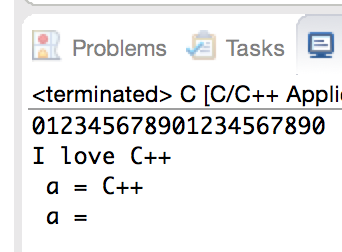
[8]문자열 검색(find() 함수)
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
cout << "012345678901234567890" << endl;
string e= "I love love C++";
cout << e << endl;
int index=e.find("love");
cout << "index = " << index << endl;
index=e.find("love", index+1);
cout << "index = " << index << endl;
index=e.find("C#");
cout << "index = " << index << endl;
index=e.find('v', 7);
cout << "index = " << index << endl;
}
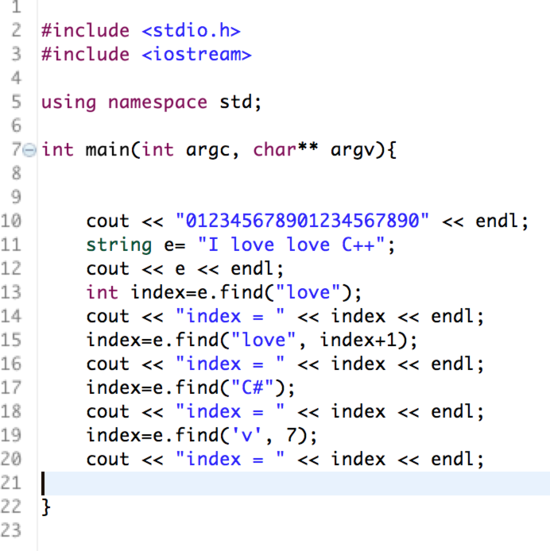
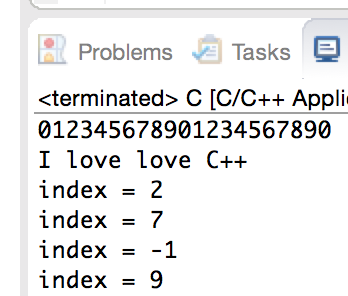
[9]특정위치 문자추출
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
cout << "012345678901234567890" << endl;
string f("I love C++");
cout << f << endl;
char ch1=f.at(7);
char ch2=f[7];
cout << "ch1 = " << ch1 << ", ch2 = " << ch2 << endl;
}
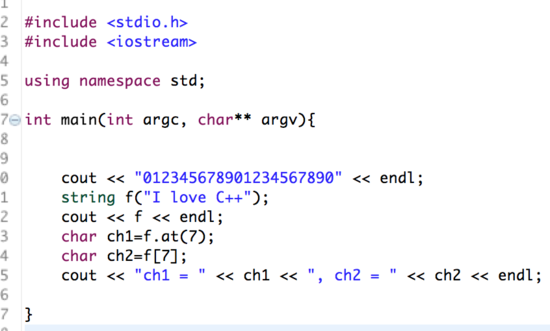
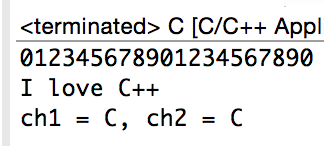
[10]문자열의 숫자 변환 stoi()
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
string year="2014";
cout << year << endl;
int n=atoi(year.c_str());
cout << n << endl;
}
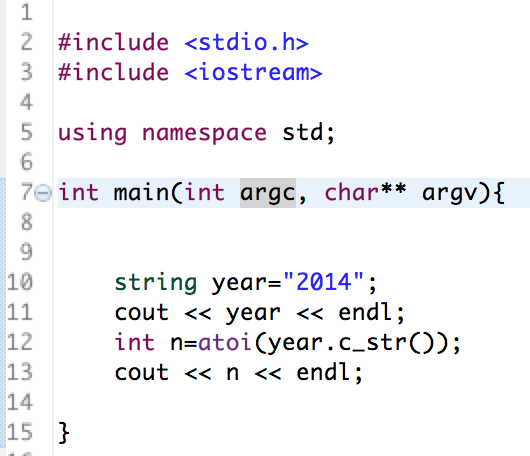
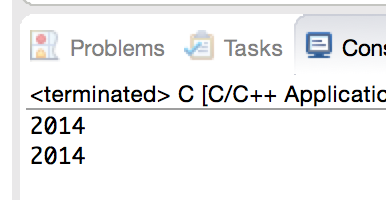
[11]문자 함수
#include <stdio.h>
#include <iostream>
using namespace std;
int main(int argc, char** argv){
string a="hello";
for(int i=0;i<a.length();i++)
a[i]=toupper(a[i]);
cout << a;
}
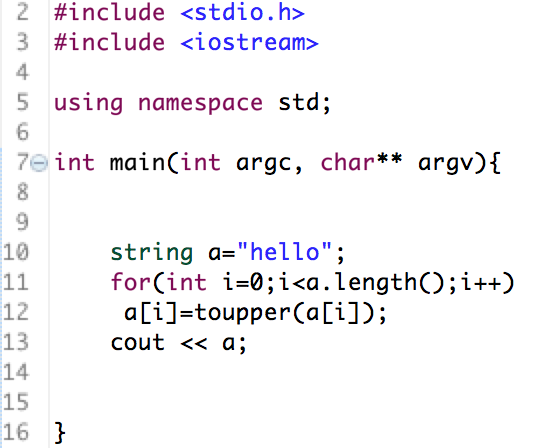
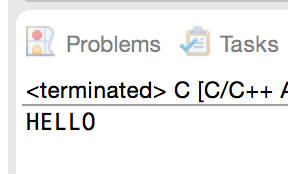
9. Person클래스를 이용한 정보검색프로그램(교재 p207, 실습문제 7번)
소스코드, 실행결과, 주석
#include <cstdlib>
#include <stdio.h>
#include <iostream>
using namespace std;
class Person{
string name;
string tel;
public:
Person();
string getName(){ return name; }
string getTel(){ return tel; }
void set(string name, string tel);
};
Person::Person() { //생성자함수 - 초기값지정
this->name="";
this->tel="";
}
void Person::set(string name, string tel){
this->name=name;
this->tel = tel;
}
class PersonManager{
Person *p;
public:
PersonManager(int n){
p=new Person[n];
string name, tel;
cout << "이름과 전화번호를 입력해 주세요" << endl;
for(int i=0;i<3;i++){
cout << "사람 " << i+1 << " : ";
cin >> name >> tel;
p[i].set(name, tel);
}
}
void show(){
cout << "모든 사람의 이름은 " << endl;
for(int i=0;i<3;i++){
cout << p[i].getName() << endl;
}
cout << endl;
}
void search(){
string name;
cout << "전화번호 검색합니다. 이름을 입력하세요 >> ";
cin >> name;
for(int i=0;i<3;i++){
if(name==p[i].getName()){
cout << "전화번호는 " << p[i].getTel() << endl;
return;
}
}
cout << "없는 사람입니다. "<< endl;
}
~PersonManager(){ //소멸자 구현
delete [] p;
}
};
int main(int argc, char *argv[]){
PersonManager manager(3);
manager.show();
manager.search();
return 0;
}
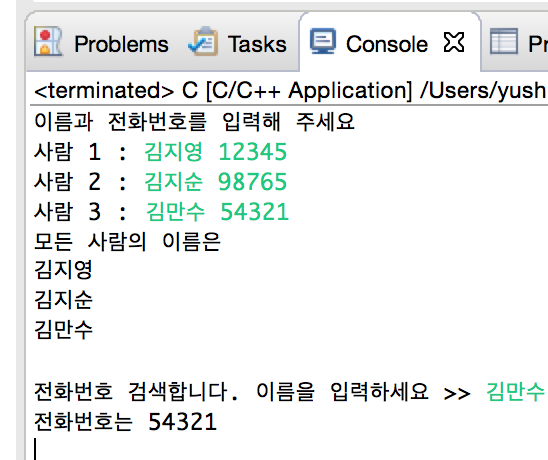
댓글
댓글 쓰기